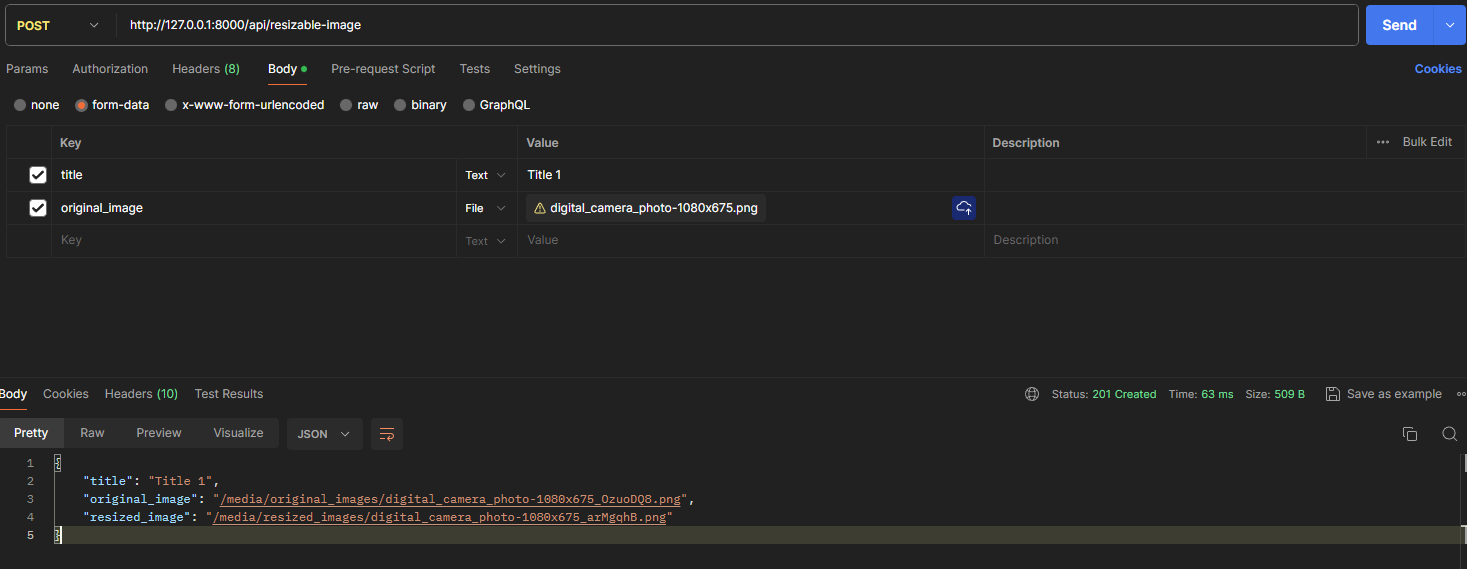
Django provides a versatile and secure approach for image uploading through Django Rest Framework (DRF). Two commonly used methods for image uploading in DRF are
- multipart/form-data:
- Base64
Setup django project for image uploading
Install required packages:
Make sure you have Django and Django Rest Framework installed:
pip install django djangorestframework
Configure Django settings:
Add 'rest_framework' and 'myapp' (replace with your app name) to INSTALLED_APPS in your settings.py:
# yourprojectname/settings.py
INSTALLED_APPS = [
# ...
'rest_framework',
'myapp',
]
Configure Django MEDIA_ROOT and MEDIA_URL:
Configure your Django settings to define the MEDIA_ROOT and MEDIA_URL, specifying where uploaded media files will be stored and how they will be accessed.
# yourproject/settings.py
MEDIA_URL = "/media/"
MEDIA_ROOT = os.path.join(BASE_DIR, "media")
Model for Image Upload:
Define a model in your models.py file to handle image uploads. Use ImageField for storing images:
# yourappname/models.py
from django.db import models
class ResizableImage(models.Model):
title = models.CharField(max_length=255)
original_image = models.ImageField(upload_to='original_images/')
resized_image = models.ImageField(upload_to='resized_images/', null=True, blank=True)
Don't forget to run migrations:
python manage.py makemigrations
python manage.py migrate
Serializer:
Create a serializer in your serializers.py file to convert your model instances to JSON and vice versa:
# yourappname/serializers.py
from rest_framework import serializers
from myapp.models import ResizableImage
from PIL import Image
from io import BytesIO
from django.core.files.base import ContentFile
class ResizableImageSerializer(serializers.ModelSerializer):
class Meta:
model = ResizableImage
fields = ('title', 'original_image', 'resized_image')
def create(self, validated_data):
print(validated_data)
instance = super().create(validated_data)
original_image = validated_data.pop('original_image')
# Resize the image
resized_image = self.resize_image(original_image)
instance.resized_image.save(original_image.name, ContentFile(resized_image), save=False)
instance.save()
return instance
def resize_image(self, image):
img = Image.open(image)
# Resize the image to a desired size (e.g., 300x300)
resized_img = img.resize((300, 300), resample=Image.BICUBIC)
# Convert the resized image to bytes
buffer = BytesIO()
resized_img.save(buffer, format='png')
img_bytes = buffer.getvalue()
return img_bytes
Views:
Create a view in your views.py file to handle image uploads:
# yourappname/views.py
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework import status
from .serializers import ResizableImageSerializer
from .serializers import ResizableImageSerializer
class ResizableImageAPIView(APIView):
def post(self, request, *args, **kwargs):
serializer = ResizableImageSerializer(data=request.data)
if serializer.is_valid():
serializer.save()
return Response(serializer.data, status=status.HTTP_201_CREATED)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
URL Configuration:
Configure your URLs in urls.py to include the DRF view:
# yourappname/urls.py
from myapp.api.views import (
ResizableImageAPIView
)
urlpatterns = [
path("api/resizable-image", ResizableImageAPIView.as_view(), name="resizable-image")
]
Runserver:
Run the development server:
python manage.py runserver
Visit http://127.0.0.1:8000/api/resizable-image in your browser or use a tool like curl or Postman to send POST requests with image files.