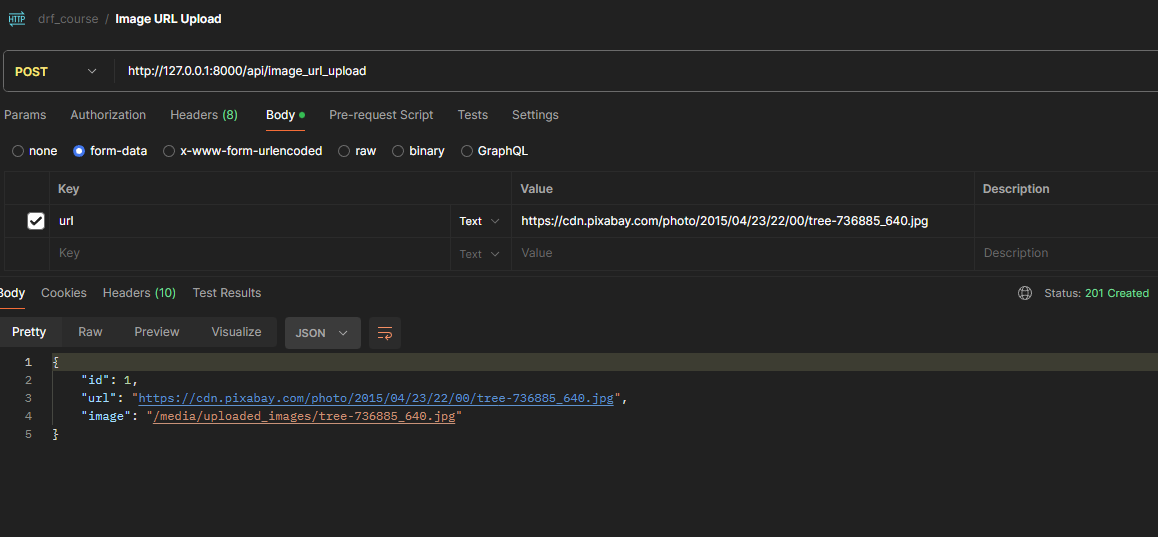
Welcome to our comprehensive guide on 'Image Upload by Uploading URL of Image.' In this article, we delve into the efficient and streamlined process of integrating images into your web applications directly from URLs. With the increasing demand for visually appealing content on the web, this method offers a convenient solution for developers to seamlessly incorporate images from external sources. Whether it's sourcing product images for an e-commerce website or aggregating user-generated content, uploading images via URL streamlines the process and reduces the need for manual downloads and uploads. Join us as we explore the ins and outs of this innovative approach, empowering you to enhance your web applications with ease
Work Flow:
- Upload the URL to the server.
- Download the image on the server from the URL
- Save images in storage and databases.
Model for Image Upload:
Define a model in your models.py file to handle image uploads. Use ImageField for storing images:
# yourappname/models.py
from django.db import models
class ImageURLUpload(models.Model):
url = models.URLField(unique=True)
image = models.ImageField(upload_to='uploaded_images/', null=True, blank=True)
Don't forget to run migrations:
python manage.py makemigrations
python manage.py migrate
Serializer:
Serializer is the best place for downloading and saving images. There are two steps:
- First, get the image URL and download the image using the Python request library.
- Save the image in storage.
- Also save in model
# yourappname/serializers.py
from rest_framework import serializers
from myapp.models import ImageURLUpload
from django.core.files import File
import requests
from io import BytesIO
class ImageURLUploadSerializer(serializers.ModelSerializer):
class Meta:
model = ImageURLUpload
fields = ('id', 'url', 'image')
def create(self, validated_data):
url = validated_data['url']
response = requests.get(url)
if response.status_code == 200:
img_data = BytesIO(response.content)
validated_data['image'] = File(img_data, name=url.split('/')[-1])
return super().create(validated_data)
Views:
Create a view in your views.py file to handle image uploads:
from rest_framework.decorators import api_view
from rest_framework.response import Response
from rest_framework import status
from myapp.models import ImageURLUpload
from .serializers import ImageURLUploadSerializer
@api_view(['POST'])
def image_url_upload_view(request):
if request.method == 'POST':
serializer = ImageURLUploadSerializer(data=request.data)
if serializer.is_valid():
serializer.save()
return Response(serializer.data, status=status.HTTP_201_CREATED)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
URL Configuration:
Configure your URLs in urls.py to include the DRF view:
# yourappname/urls.py
from django.urls import path
from myapp.api.views import image_url_upload_view
urlpatterns = [
path('api/image_url_upload', image_url_upload_view, name='image-upload'),
# Add more patterns as needed
]